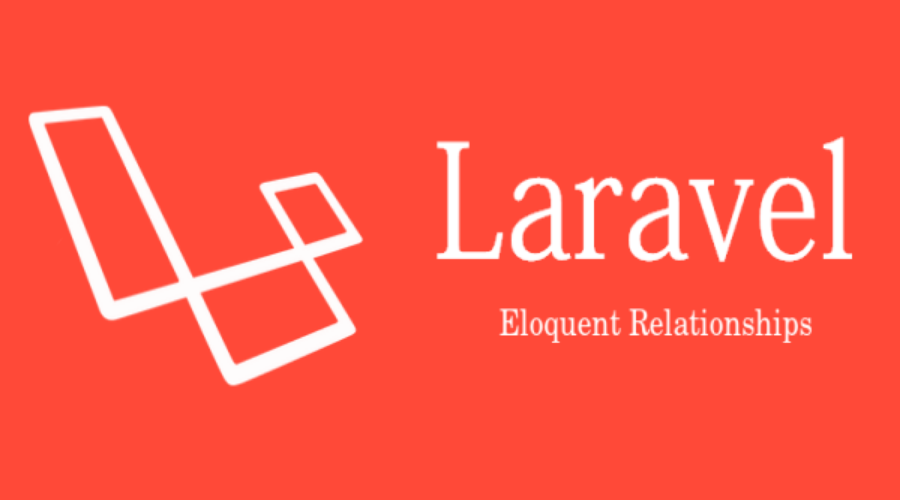
In part I, we created database tables, models and defined relationships. Let’s now see how relationships make dev a breeze. For the purpose of demonstrating this, create a controller by running the command php artisan make:controller TestController. You can name yours however you like and a route
Route::get('/posts', 'TestController@getPosts');
One big win when you use Laravel relationships is limiting the number of queries you make to the database by preventing the n+1 problem. Assuming we did not use relationships and we want data about all the posts, their comments and those who posted them! We would do something like:
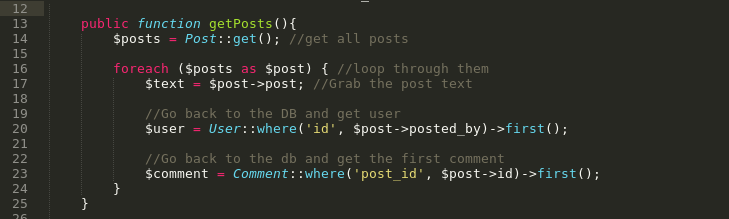
As you can see for every post we have on the database, we are going back to the database twice to fetch the the user and comments. Every developer strives to limit trips to the database as much as possible. Laravel relationships allow us to solve this problem using lazy loading. To get all the post, their posts and comments, we do this:
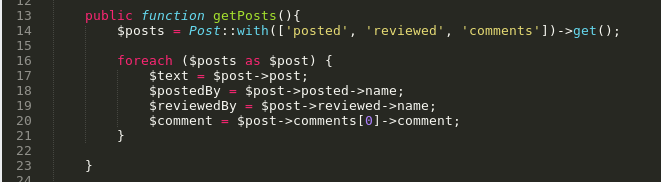
Here we grab all the posts with who posted them, who reviewed them, and their comments – with a single trip to the database! I added the 0 constrain to the comments since they are many, otherwise to access each comment, you have to loop through them. Notice the ‘with’ clause uses the relationships we defined in the Post model (posted, reviewed, comments). We also use the same when accessing the data in the loop. You can also apply constraints to the relationships. Assume you want the last 5 posts, with who posted them, who reviewed them and their first 10 comments. Your query will look like this:
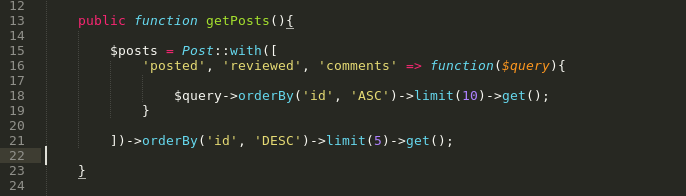
We applied constraints to the Post model as well as Comment model. You can apply all sorts of constraints like where, whereIn, groupBy etc.
With Blade Template
Lastly let’s print in a view the 5 posts we grabbed above, with the name of who posted, who reviewed and also their first 10 comments.
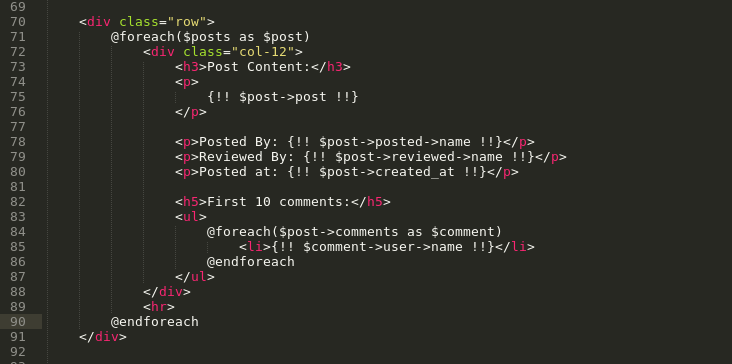
That’s it. Happy coding!